Sign In with Apple
When integrating third-party sign-in methods on an iOS app, it is mandatory to add the sign in with Apple option as an additional alternative. For this purpose, Apple introduced the AuthenticationServices framework. This framework allows developers to seamlessly integrate a Sign in with Apple button on any Xcode project.
In this repository we provide a simple Xcode template where you can test the different sign in methods we are implementing. This example was already introduced in the log in guide. You can revisit it for more details about the project.
To complete this quickstart, you need:
- A recent version of Xcode.
- An Apple developer account with non-personal developer team.
- An app created at Back4App.
- Note: Follow the Install Parse SDK (Swift) Tutorial to create an Xcode Project connected to Back4App.
To integrate a user sign-in feature using the AuthenticationServices framework and ParseSwift SDK.
- Once we have the Xcode project linked to the Back4App application, we proceed to add the Sign in with Apple capability. To do this, select your project from the project navigator and go to the targets section. Select the main target and go to the Signing & Capabilities tab, then click on the + Capability button and add the Sign in with Apple capability:
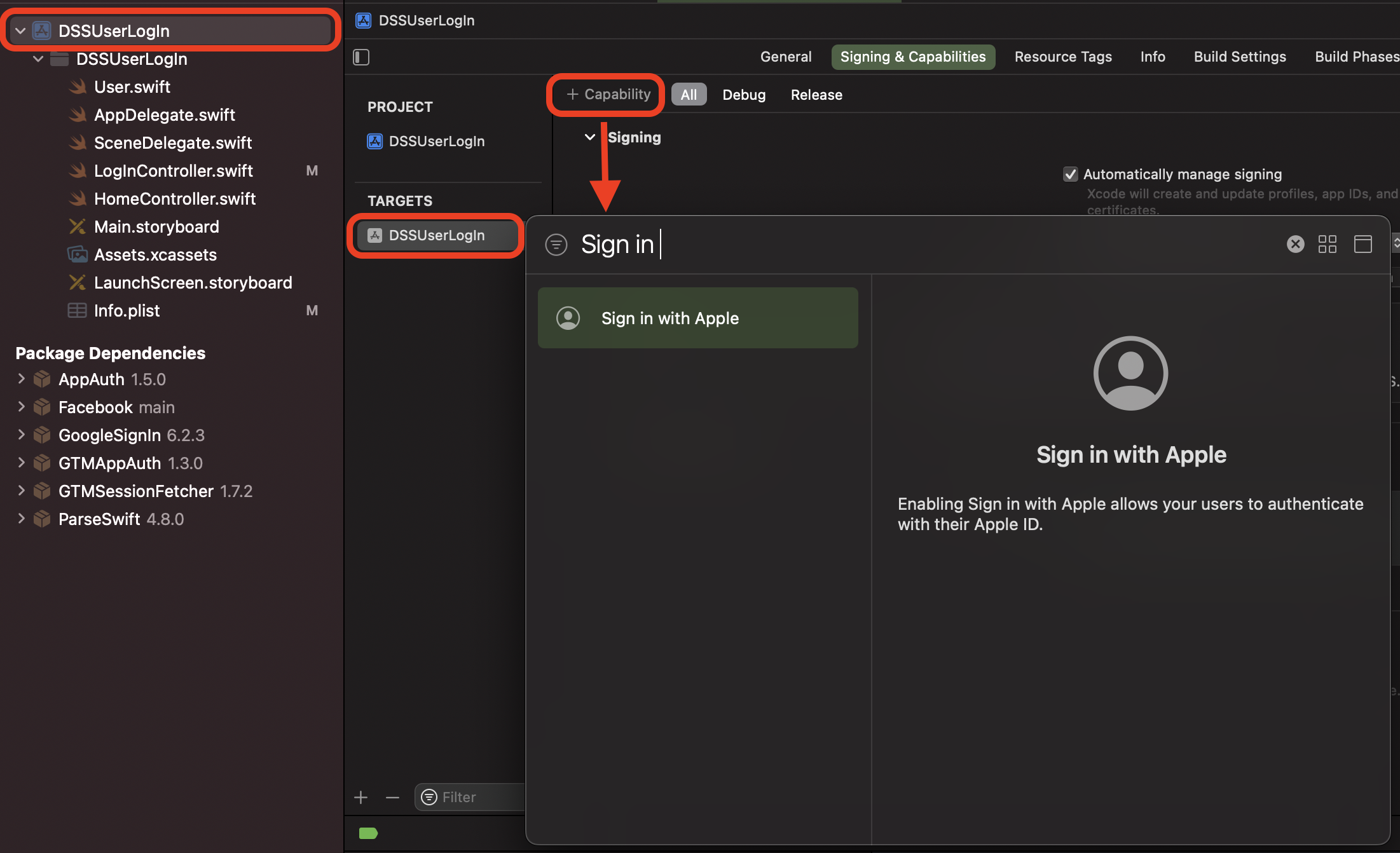
This is the only configuration needed to start integrating a Sign in with Apple method on an iOS app.
The sign in with Apple flow can be completed in three stages. But before, let us add and set up the button to be used to present the Sign in with Apple flow. In the LogInControllerclass we add this button:
Note that the LogInController conforms to two new protocols: ASAuthorizationControllerDelegate and ASAuthorizationControllerPresentationContextProviding. The first protocol allows us to delegate the sign-in result to the LogInController class. The second protocol is to determine the UIWindow where the sign-in sheet is displayed.
We now implement the flow in the handleSignInWithApple() method.
- In the first stage, we prepare the request and the form. The request is constructed by the ASAuthorizationAppleIDRequest class. We get an instance of this class from the ASAuthorizationAppleIDProvider provider and the form by the ASAuthorizationController class. Once we have an instance of the request, we have to provide the scopes we are interested in. So far Apple only gives access to the user’s full name and email. Thus, a standard way to create a request is:
- With this request, we construct an ASAuthorizationController controller. This controller is in charge of displaying a sheet where the user authenticates and gives the corresponding permissions to complete the sign-in process:
- In the last stage we handle the sign-in result. This is returned via the delegate method authorizationController(controller:didCompleteWithAuthorization:). The last argument in this method is an ASAuthorization class containing all the necessary information about the Apple ID and credentials. This stage is where we associate a User object and perform the log in to the Back4App application. This User object has the following structure (see the Login guide for more details):
Now, we create a User object from the data contained in the ASAuthorization result. We accomplish this by instantiating a ParseApple object (from User.apple) and call the login(user:identityToken:) method:
To make sure that the Google sign-in worked, you can look at your Back4App application dashboard and see the new User containing the Facebook authData parameters.
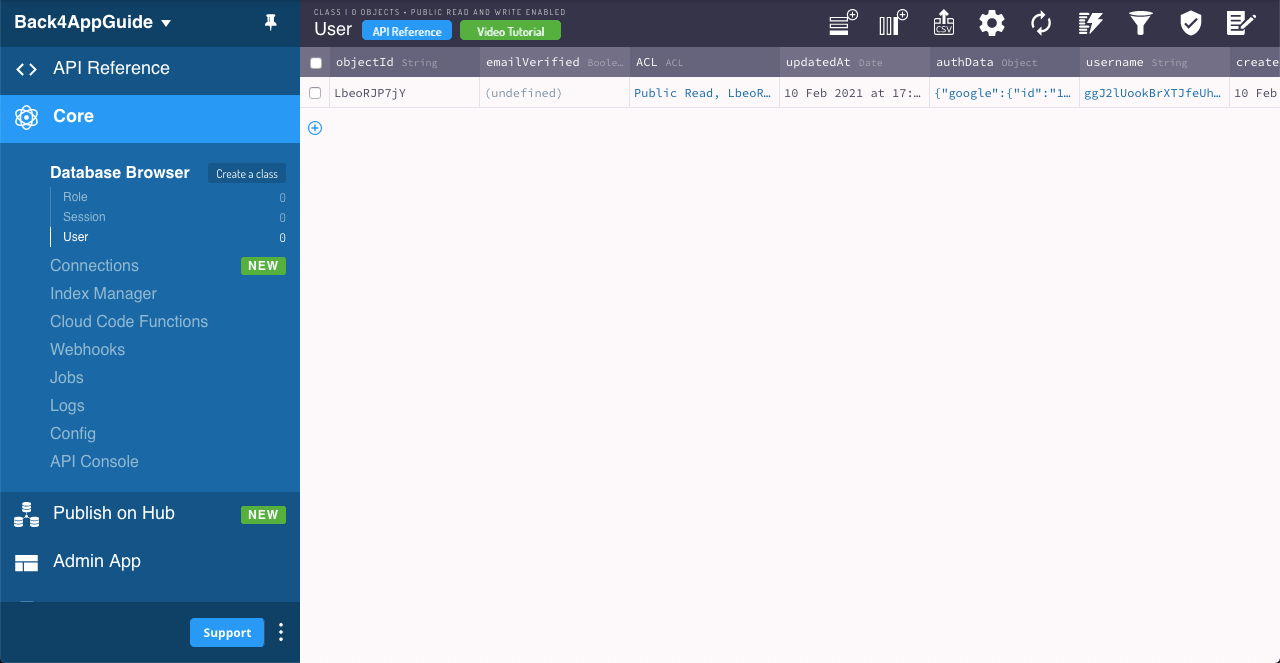
You can also verify that a valid session was created in the dashboard, containing a pointer to the corresponding User object.
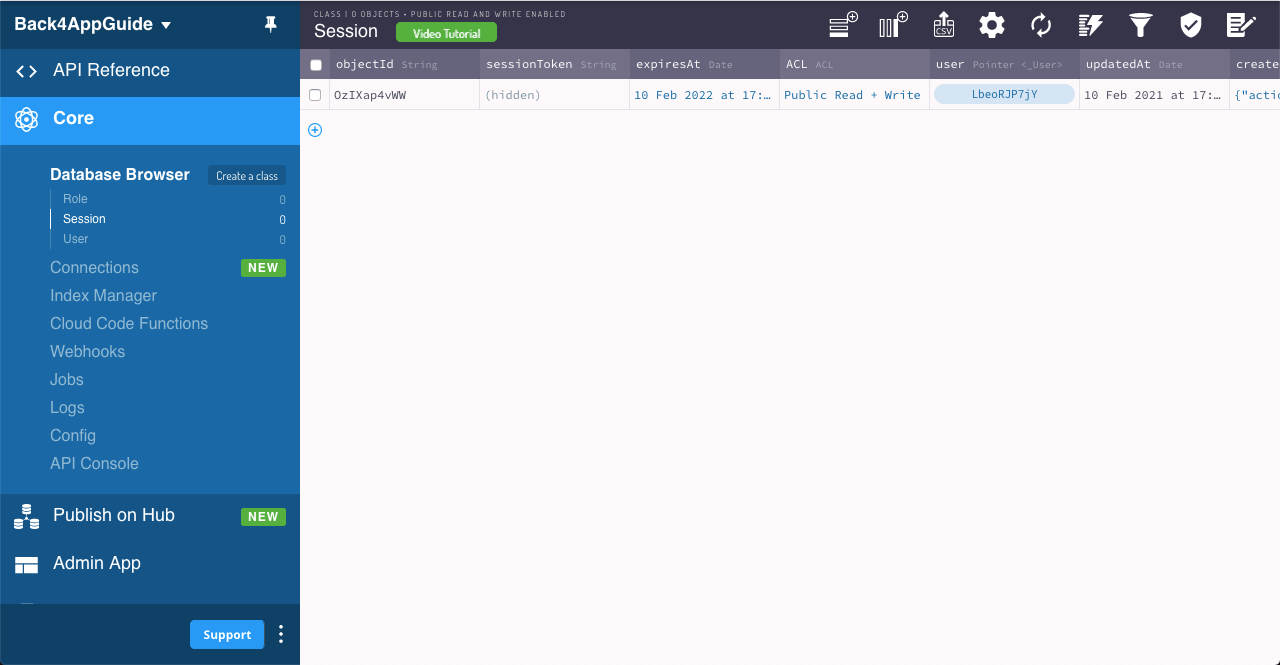
In case your iOS App requires relating an Apple ID to an existing user in your Back4App platform, the ParseApple<User> object implements the method link(user:identityToken:completion:) where you pass the user value and the identityToken from a ASAuthorizationAppleIDCredential credential
You can go to this repository and download the example project. Before running the project, make sure you set up the provisioning profiles with the ones associated with your developer account.
At the end of this guide, you learned how to sign in or link existing Back4App users on iOS using the sign in with Apple.