Basic Queries
In most use cases, we require to fetch data from a database with certain conditions. These conditions may include complex comparisons and ordering requirements. Thus, in any application, it is fundamental to construct efficient queries and, at the same time, the database has to be able to execute them as fast as possible.
The ParseSwift SDK does provide the necessary tools for you to construct any query according to the application requirements. In this tutorial, we explore these tools and use them in a real-world application.
This tutorial uses a basic app created in Xcode 12 and iOS 14.
At any time, you can access the complete Project via our GitHub repositories.
- To understand how to create basic queries to retrieve data from a Back4App Database.
To complete this quickstart, you need:
- Xcode.
- An app created at Back4App.
- Note: Follow the Install Parse SDK (Swift) Tutorial to create an Xcode Project connected to Back4App.
The project template is a Contacts App where the user adds a contact’s information to save it on a Back4App Database
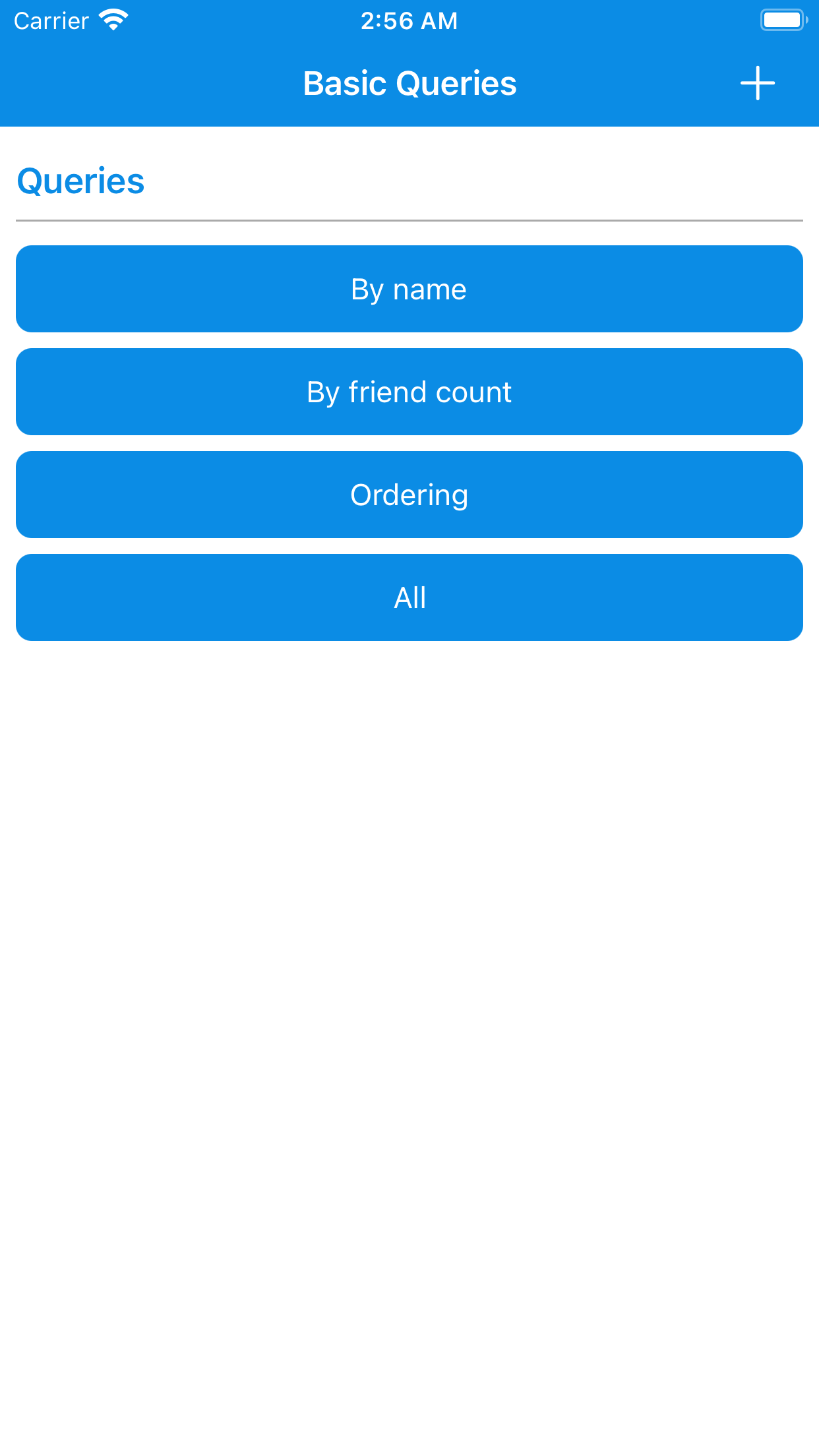
On the app’s homescreen you will find a set of buttons for different types of queries. Using the + button located on the top-right side of the navigation bar, we can add as many Contacts as needed.
For this example, we use the object Contact
The following methods will allows us to save and queryContactobjects:
The XCode project has the following structure
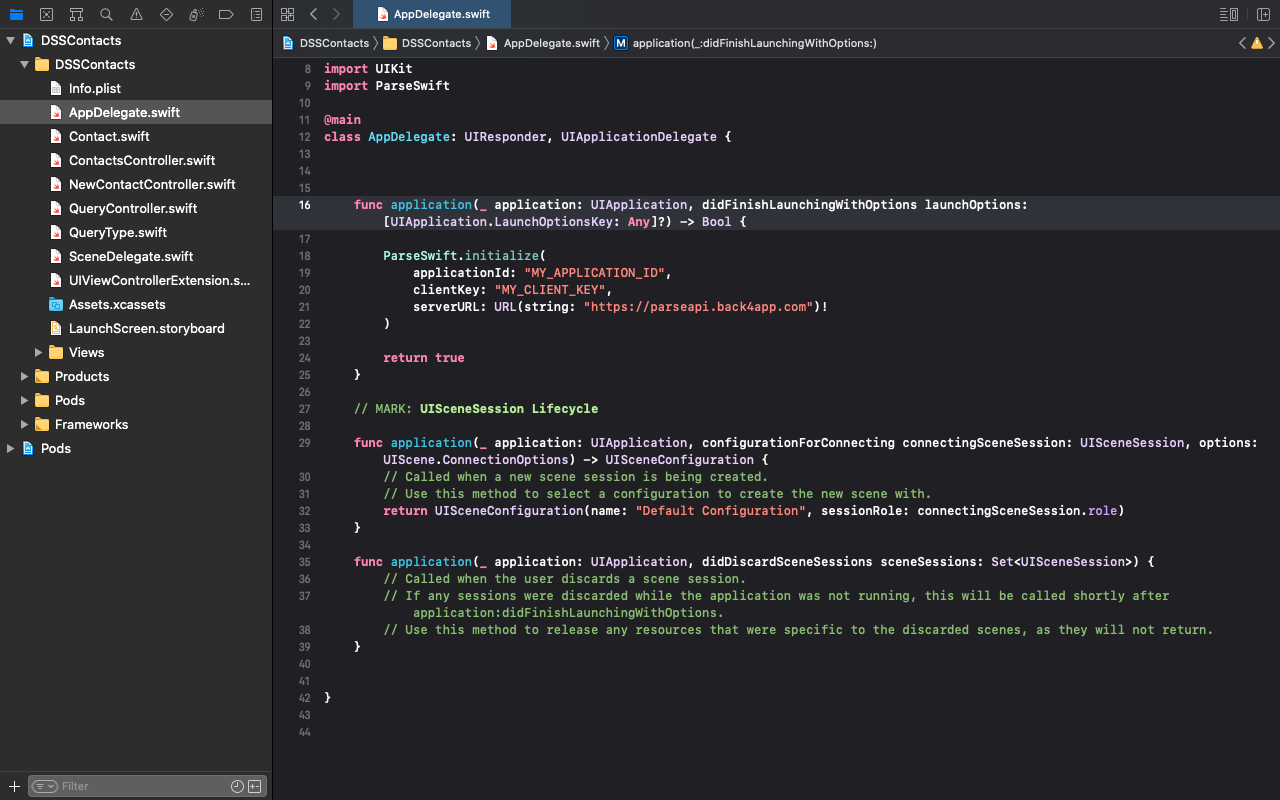
At any time, you can access the complete Project via our GitHub repositories.
To focus on the main objective of this guide, we will only detail the sections strictly related to queries and the ParseSwift SDK.
Before getting started with queries, it is necessary to have some contacts already saved on your Back4App Database. In the NewContactController class, we implement a basic form to add a Contact. To save an instance of a Contact object, we use the handleAddContact() method implemented in the NewContactController class
For more details about this step, you can go to the basic operations guide.
- By name
The first example we look at is a query that allows us to retrieve contacts which have a specific substring in their name field. In order to do this, we first create a QueryConstraint object. This object will contain the constraint we want. The ParseSwift SDK provides the following methods to (indirectly) create a QueryConstraint
For instance, a query that allows us to retrieve all the Contact’s with John in their name field can be created with
In case the constraint requires the name field to match exactly a given string, we can use
- By number of friends
A query with a constraint involving a numerical comparison can be constructed by creating aQueryConstraint with
To query all contacts with 30 or more friends, we use
- Ordering query results
For ordering the results from a query, the Query<contacts> object provides the method order(_:) which returns a new Query<contact> object considering the requested ordering option. As a parameter, we pass an enumeration (Query<contact>.Order) to indicate the ordering we want. The following snippet applies a descending order based on the birthday field
In the project example, we implemented the queries mentioned above. The ContactsController class has the method fetchContacts() where you will find the following snippet
Before pressing the run button on XCode, do not forget to configure your Back4App application in the AppDelegate class!
Using the+button in the navigation bar, add a counple of contacts and test the different queries.